mirror of
https://bitbucket.org/Ioncannon/project-meteor-server.git
synced 2025-07-15 19:07:44 +00:00
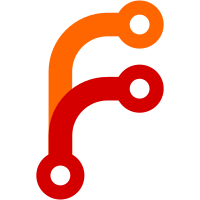
- AddItem functions to cast INV_ERROR to INT for LUA - Fixed unique item check. It was checking for Rare flag, not EX Scripts : Base - shop.lua : Functions for buying/selling from a variety of shop scripts Commands - EmoteStandardCommand.lua fixed not being able to use emotes when sitting - DiceCommand.lua fixed. No arguments sets default value of 100 as per the ingame description. Max value raised from 999 to 1000. GM Commands - speed.lua fixed when using single argument - nudge.lua fixed sanitizing. Made arguments reversible to allow !nudge up 10 & !nudge 10 up - giveitem.lua added inv_error handling. Need to do to rest of item commands at some point - givecurrency.lua changed to have you enter a regex'd name of item rather than item ID. Eg. "!givecurrency fire_crystal 10". Added inv_error handling to it. - warpplayer.lua added. Moves yourself to name of player, or moves first player to second player - warpid.lua added. For warping to the first instance of an actor's uniqueId the server comes across. - quest.lua added. For adding/adjusting quests for debugging them. Class Scripts - PopulaceBlackMarketeer.lua updated to utilize shop.lua - PopulaceShopSalesman.lua updated to utilize shop.lua - PopulaceCompanyShop.lua updated to utilize shop.lua - PopulaceCompanyBuffer.lua added and documented along with menu layout. Needs working status effect to finish. - PopulaceCompanyGLPublisher.lua added. Mostly documented, barely functional. - PopulaceCompanyGuide.lua added. Documented, fully functional. - PopulaceCompanyOfficer.lua added. Documented. Menus work. Needs GC rank table at some point for documenting GC ranks/seal caps. - PopulaceCompanySupply.lua added and mostly documented. Read-only basic menu flow, static LUA tables used to set it up, will need SQL tables at some point to replace them with. Some guesswork on what menus show since no video reference could be found. - PopulaceGuildShop.lua updated. Mostly documented. Read-only shop menus.
108 lines
No EOL
2.4 KiB
Lua
108 lines
No EOL
2.4 KiB
Lua
--[[
|
|
|
|
Globals referenced in all of the lua scripts
|
|
|
|
--]]
|
|
|
|
-- ACTOR STATES
|
|
|
|
ACTORSTATE_PASSIVE = 0;
|
|
ACTORSTATE_DEAD1 = 1;
|
|
ACTORSTATE_ACTIVE = 2;
|
|
ACTORSTATE_DEAD2 = 3;
|
|
ACTORSTATE_SITTING_ONOBJ = 11;
|
|
ACTORSTATE_SITTING_ONFLOOR = 13;
|
|
ACTORSTATE_MOUNTED = 15;
|
|
|
|
|
|
-- MESSAGE
|
|
MESSAGE_TYPE_NONE = 0;
|
|
MESSAGE_TYPE_SAY = 1;
|
|
MESSAGE_TYPE_SHOUT = 2;
|
|
MESSAGE_TYPE_TELL = 3;
|
|
MESSAGE_TYPE_PARTY = 4;
|
|
MESSAGE_TYPE_LINKSHELL1 = 5;
|
|
MESSAGE_TYPE_LINKSHELL2 = 6;
|
|
MESSAGE_TYPE_LINKSHELL3 = 7;
|
|
MESSAGE_TYPE_LINKSHELL4 = 8;
|
|
MESSAGE_TYPE_LINKSHELL5 = 9;
|
|
MESSAGE_TYPE_LINKSHELL6 = 10;
|
|
MESSAGE_TYPE_LINKSHELL7 = 11;
|
|
MESSAGE_TYPE_LINKSHELL8 = 12;
|
|
|
|
MESSAGE_TYPE_SAY_SPAM = 22;
|
|
MESSAGE_TYPE_SHOUT_SPAM = 23;
|
|
MESSAGE_TYPE_TELL_SPAM = 24;
|
|
MESSAGE_TYPE_CUSTOM_EMOTE = 25;
|
|
MESSAGE_TYPE_EMOTE_SPAM = 26;
|
|
MESSAGE_TYPE_STANDARD_EMOTE = 27;
|
|
MESSAGE_TYPE_URGENT_MESSAGE = 28;
|
|
MESSAGE_TYPE_GENERAL_INFO = 29;
|
|
MESSAGE_TYPE_SYSTEM = 32;
|
|
MESSAGE_TYPE_SYSTEM_ERROR = 33;
|
|
|
|
-- INVENTORY
|
|
INVENTORY_NORMAL = 0x0000; --Max 0xC8
|
|
INVENTORY_LOOT = 0x0004; --Max 0xA
|
|
INVENTORY_MELDREQUEST = 0x0005; --Max 0x04
|
|
INVENTORY_BAZAAR = 0x0007; --Max 0x0A
|
|
INVENTORY_CURRENCY = 0x0063; --Max 0x140
|
|
INVENTORY_KEYITEMS = 0x0064; --Max 0x500
|
|
INVENTORY_EQUIPMENT = 0x00FE; --Max 0x23
|
|
INVENTORY_EQUIPMENT_OTHERPLAYER = 0x00F9; --Max 0x23
|
|
|
|
-- INVENTORY ERRORS
|
|
INV_ERROR_SUCCESS = 0;
|
|
INV_ERROR_FULL = 1;
|
|
INV_ERROR_ALREADY_HAS_UNIQUE = 2;
|
|
INV_ERROR_SYSTEM_ERROR = 3;
|
|
|
|
-- CHOCOBO APPEARANCE
|
|
CHOCOBO_NORMAL = 0;
|
|
|
|
CHOCOBO_LIMSA1 = 0x1;
|
|
CHOCOBO_LIMSA2 = 0x2;
|
|
CHOCOBO_LIMSA3 = 0x3;
|
|
CHOCOBO_LIMSA4 = 0x4;
|
|
|
|
CHOCOBO_GRIDANIA1 = 0x1F;
|
|
CHOCOBO_GRIDANIA2 = 0x20;
|
|
CHOCOBO_GRIDANIA3 = 0x21;
|
|
CHOCOBO_GRIDANIA4 = 0x22;
|
|
|
|
CHOCOBO_ULDAH1 = 0x3D;
|
|
CHOCOBO_ULDAH2 = 0x3E;
|
|
CHOCOBO_ULDAH3 = 0x3F;
|
|
CHOCOBO_ULDAH4 = 0x40;
|
|
|
|
--UTILS
|
|
|
|
function kickEventContinue(player, actor, trigger, ...)
|
|
player:kickEvent(actor, trigger, ...);
|
|
return coroutine.yield("_WAIT_EVENT", player);
|
|
end
|
|
|
|
function callClientFunction(player, functionName, ...)
|
|
player:RunEventFunction(functionName, ...);
|
|
return coroutine.yield("_WAIT_EVENT", player);
|
|
end
|
|
|
|
function wait(seconds)
|
|
return coroutine.yield("_WAIT_TIME", seconds);
|
|
end
|
|
|
|
function waitForSignal(signal)
|
|
return coroutine.yield("_WAIT_SIGNAL", signal);
|
|
end
|
|
|
|
function sendSignal(signal)
|
|
GetLuaInstance():OnSignal(signal);
|
|
end
|
|
|
|
function printf(s, ...)
|
|
if ... then
|
|
print(s:format(...));
|
|
else
|
|
print(s);
|
|
end;
|
|
end; |