mirror of
https://bitbucket.org/Ioncannon/project-meteor-server.git
synced 2025-04-21 12:17:46 +00:00
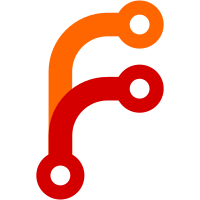
Added the combo and proc systems Added scripts for most weaponskill and spells as well as some abilities and status effects Added support for multihit attacks Added AbilityState for abilities Added hiteffects that change based on an attack's parameters Added positionals Changed how targeting works for battlecommands Fixed bug that occurred when moving or swapping hotbar commands Fixed bug that occurred when losing status effects
71 lines
No EOL
2 KiB
Lua
71 lines
No EOL
2 KiB
Lua
-- todo: add enums for status effects in global.lua
|
|
require("global")
|
|
require("battleutils")
|
|
|
|
magic =
|
|
{
|
|
|
|
};
|
|
|
|
--[[
|
|
statId - see BattleTemp.cs
|
|
modifierId - Modifier.Intelligence, Modifier.Mind (see Modifier.cs)
|
|
multiplier -
|
|
]]
|
|
function magic.HandleHealingMagic(caster, target, spell, action, statId, modifierId, multiplier, baseAmount)
|
|
potency = potency or 1.0;
|
|
healAmount = baseAmount;
|
|
|
|
-- todo: shit based on mnd
|
|
local mind = caster.GetMod(Modifier.Mind);
|
|
end;
|
|
|
|
function magic.HandleAttackMagic(caster, target, spell, action, statId, modifierId, multiplier, baseAmount)
|
|
-- todo: actually handle this
|
|
damage = baseAmount or math.random(1,10) * 10;
|
|
|
|
return damage;
|
|
end;
|
|
|
|
function magic.HandleEvasion(caster, target, spell, action, statId, modifierId)
|
|
|
|
return false;
|
|
end;
|
|
|
|
function magic.HandleStoneskin(caster, target, spell, action, statId, modifierId, damage)
|
|
--[[
|
|
if target.statusEffects.HasStatusEffect(StatusEffect.Stoneskin) then
|
|
-- todo: damage reduction
|
|
return true;
|
|
end;
|
|
]]
|
|
return false;
|
|
end;
|
|
|
|
function magic.onMagicFinish(caster, target, spell, action)
|
|
action.battleActionType = BattleActionType.AttackMagic;
|
|
local damage = math.random(50, 150);
|
|
action.amount = damage;
|
|
action.CalcHitType(caster, target, spell);
|
|
action.TryStatus(caster, target, spell, true);
|
|
return action.amount;
|
|
end;
|
|
|
|
--For healing magic
|
|
function magic.onCureMagicFinish(caster, target, spell, action)
|
|
action.battleActionType = BattleActionType.Heal;
|
|
local amount = math.random(200, 450);
|
|
action.amount = amount;
|
|
action.CalcHitType(caster, target, spell);
|
|
action.TryStatus(caster, target, spell, true);
|
|
return action.amount;
|
|
end;
|
|
|
|
--For status magic
|
|
function magic.onStatusMagicFinish(caster, target, spell, action)
|
|
action.battleActionType = BattleActionType.Status;
|
|
action.amount = 0;
|
|
action.CalcHitType(caster, target, spell);
|
|
action.TryStatus(caster, target, spell, false);
|
|
return action.amount;
|
|
end; |