mirror of
https://bitbucket.org/Ioncannon/project-meteor-server.git
synced 2025-04-22 12:47:46 +00:00
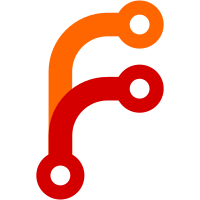
- regex'd in mysqlexception logging - servers can now specify server_port, log_path, log_file - added scripts to import/export all tables (exporting will export a handful of garbage table names, open and check for structure before deleting) - fixed packet logging (thanks deviltti)
67 lines
1.9 KiB
C#
67 lines
1.9 KiB
C#
using System.Collections.Generic;
|
|
|
|
namespace FFXIVClassic_Map_Server.actors
|
|
{
|
|
class EventList
|
|
{
|
|
public List<TalkEventCondition> talkEventConditions;
|
|
public List<NoticeEventCondition> noticeEventConditions;
|
|
public List<EmoteEventCondition> emoteEventConditions;
|
|
public List<PushCircleEventCondition> pushWithCircleEventConditions;
|
|
public List<PushFanEventCondition> pushWithFanEventConditions;
|
|
public List<PushBoxEventCondition> pushWithBoxEventConditions;
|
|
|
|
public class TalkEventCondition
|
|
{
|
|
public byte unknown1;
|
|
public bool isDisabled = false;
|
|
public string conditionName;
|
|
}
|
|
|
|
public class NoticeEventCondition
|
|
{
|
|
public byte unknown1;
|
|
public byte unknown2;
|
|
public string conditionName;
|
|
|
|
public NoticeEventCondition(string name, byte unk1, byte unk2)
|
|
{
|
|
conditionName = name;
|
|
unknown1 = unk1;
|
|
unknown2 = unk2;
|
|
}
|
|
}
|
|
|
|
public class EmoteEventCondition
|
|
{
|
|
public byte unknown1;
|
|
public byte unknown2;
|
|
public byte emoteId;
|
|
public string conditionName;
|
|
}
|
|
|
|
public class PushCircleEventCondition
|
|
{
|
|
public string conditionName = "";
|
|
public float radius = 30.0f;
|
|
public bool outwards = false;
|
|
public bool silent = true;
|
|
}
|
|
|
|
public class PushFanEventCondition
|
|
{
|
|
public string conditionName;
|
|
public float radius = 30.0f;
|
|
public bool outwards = false;
|
|
public bool silent = true;
|
|
}
|
|
|
|
public class PushBoxEventCondition
|
|
{
|
|
public string conditionName = "";
|
|
public float size = 30.0f;
|
|
public bool outwards = false;
|
|
public bool silent = true;
|
|
}
|
|
}
|
|
}
|