mirror of
https://bitbucket.org/Ioncannon/project-meteor-server.git
synced 2025-07-09 00:17:46 +00:00
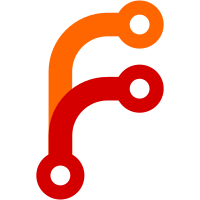
- renamed sln to FFXIVClassic.sln - threaded logging - todo: print packets using Log.Packet
74 lines
No EOL
2.2 KiB
C#
74 lines
No EOL
2.2 KiB
C#
using System;
|
|
|
|
namespace FFXIVClassic.Common
|
|
{
|
|
[global::System.AttributeUsage(AttributeTargets.Field, AllowMultiple = false)]
|
|
public sealed class BitfieldLengthAttribute : Attribute
|
|
{
|
|
uint length;
|
|
|
|
public BitfieldLengthAttribute(uint length)
|
|
{
|
|
this.length = length;
|
|
}
|
|
|
|
public uint Length { get { return length; } }
|
|
}
|
|
|
|
public static class PrimitiveConversion
|
|
{
|
|
public static UInt32 ToUInt32<T>(T t) where T : struct
|
|
{
|
|
UInt32 r = 0;
|
|
int offset = 0;
|
|
|
|
// For every field suitably attributed with a BitfieldLength
|
|
foreach (System.Reflection.FieldInfo f in t.GetType().GetFields())
|
|
{
|
|
object[] attrs = f.GetCustomAttributes(typeof(BitfieldLengthAttribute), false);
|
|
if (attrs.Length == 1)
|
|
{
|
|
uint fieldLength = ((BitfieldLengthAttribute)attrs[0]).Length;
|
|
|
|
// Calculate a bitmask of the desired length
|
|
uint mask = 0;
|
|
for (int i = 0; i < fieldLength; i++)
|
|
mask |= (UInt32)1 << i;
|
|
|
|
r |= ((UInt32)f.GetValue(t) & mask) << offset;
|
|
|
|
offset += (int)fieldLength;
|
|
}
|
|
}
|
|
|
|
return r;
|
|
}
|
|
|
|
public static long ToLong<T>(T t) where T : struct
|
|
{
|
|
long r = 0;
|
|
int offset = 0;
|
|
|
|
// For every field suitably attributed with a BitfieldLength
|
|
foreach (System.Reflection.FieldInfo f in t.GetType().GetFields())
|
|
{
|
|
object[] attrs = f.GetCustomAttributes(typeof(BitfieldLengthAttribute), false);
|
|
if (attrs.Length == 1)
|
|
{
|
|
uint fieldLength = ((BitfieldLengthAttribute)attrs[0]).Length;
|
|
|
|
// Calculate a bitmask of the desired length
|
|
long mask = 0;
|
|
for (int i = 0; i < fieldLength; i++)
|
|
mask |= 1 << i;
|
|
|
|
r |= ((UInt32)f.GetValue(t) & mask) << offset;
|
|
|
|
offset += (int)fieldLength;
|
|
}
|
|
}
|
|
|
|
return r;
|
|
}
|
|
}
|
|
} |